Best Practices in API Design
Table Of Content

The secret sauce that elevates your RESTful API from good to great is performance optimization. From investing in reliable and fast network infrastructure to tracking various aspects of an API, every detail contributes to the performance of your API. When there are multiple rows of data available then APIs should give the requested data in batch-wise (Pagination). Design for intent is a method that expresses the different relationships between objects so that changes to one object automatically propagates changes to others.
function handleChange()
We've already implemented the endpoints correctly without using verbs inside the URL, but let's take a look how our URL's would look like if we had used verbs. It doesn't make much sense to use verbs inside your endpoints and is, in fact, pretty useless. Generally each URL should point towards a resource (remember the box example from above). Leaving this error message more generic for all properties will be okay for now.
Architecture
The above REST API design patterns help create simple, scalable, and easy-to-maintain APIs. REST (Representational State Transfer) API design patterns follow best practices and guidelines for web services. If we try to add a new workout but forget to provide the "mode" property in our request body, we should see the error message along with the 400 HTTP error code. When something goes wrong (either from the request or inside our API) we send HTTP Error codes back. I've seen and used API's that were returning all the time a 400 error code when a request was buggy without any specific message about WHY this error occurred or what the mistake was.

$(".ownership-indicator").addClass('owned');
The gateway acts as a first line of defense, enforcing security policies, access controls, and threat mitigation strategies to safeguard the microservices ecosystem. Gateway offloading is a practice in microservices architecture where certain tasks or responsibilities are shifted away from the individual microservices and delegated to a centralized gateway or proxy. This offloading helps optimize the performance and scalability of the microservices ecosystem by reducing the burden on individual services. Imagine you’re building an e-commerce platform where you need to integrate various services and functionalities from different providers to offer a comprehensive shopping experience. These functionalities may include product listings, payment processing, order tracking, and user authentication.
Use Cases of Gateway Routing:
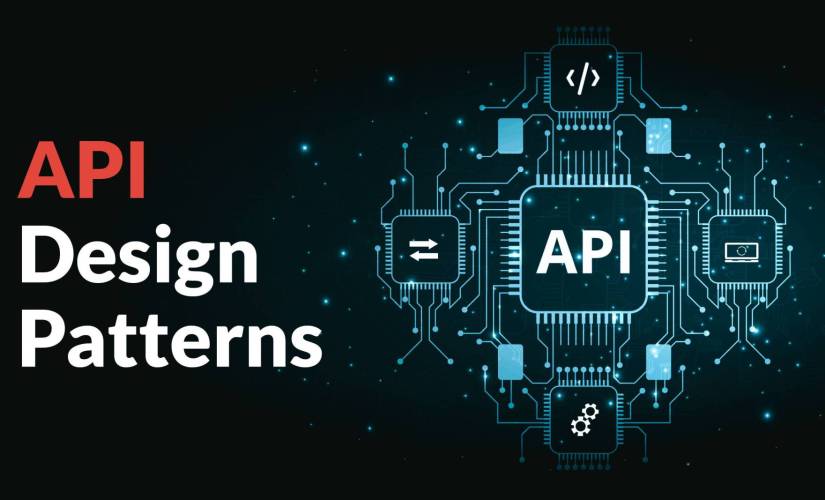
We hope you find theintermediate results of our ongoing efforts useful. We will be glad tohear about your feedback and constructive criticism. We also welcomecontributions such as pointers to known uses or war stories in which youhave seen some of the patterns (or variants of them) in action. Microservices architectures have evolved from previous incarnationsof Service-Oriented Architectures (SOAs). They consistof independently deployable, scalable and changeable services, eachhaving a single responsibility. Microservices often aredeployed in lightweight virtualization containers, encapsulate their ownstate, and communicate via message-based remote APIs in a loosely coupled fashion.
Software Application Architecture
The endpoint for creating or updating a workout needs data from the client. In other words, let's start implementing endpoints for creating, reading, updating and deleting workouts. You've just structured the project for handling different versions.
When a user requests to view product details, the gateway inspects the request and forwards it to the product catalog service. Similarly, a request to place an order is routed to the order management service. In a microservices architecture, each service has its process and communicates with the other services by a well-defined API which is built on the top of a network. We can add caching to return data from the local memory cache instead of querying the database to get the data every time we want to retrieve some data that users request.
Emerging Architectures for LLM Applications - Andreessen Horowitz
Emerging Architectures for LLM Applications.
Posted: Tue, 20 Jun 2023 07:00:00 GMT [source]
But as you might know, in the real world a lot of errors can happen – either from a human or a technical perspective. Our API has the ability now to handle basic CRUD operations with data storage. First, we create a simple Util Function to overwrite our JSON file to persist the data. Jump right back into our workout service and implement the logic for getAllWorkouts. One workout consists of an id, name, mode, equipment, exercises, createdAt, updatedAt, and trainerTips. Let's take a look at our current implementation and see how we can integrate this best practice.
API security design best practices for enterprise and public cloud - Хабр
API security design best practices for enterprise and public cloud.
Posted: Mon, 13 Dec 2021 08:00:00 GMT [source]
As you’re designing RESTful APIs, you’ll want to rely on the HTTP methods and best practices to express the primary purpose of a call. For that reason, you don’t want to use a POST to simply retrieve data. You should examine your use cases to determine when to use each.
Sometimes, there's so much data that it shouldn’t be returned all at once because it’s way too slow or will bring down our systems. Whenever our API does not successfully complete, we should fail gracefully by sending an error with information to help users make corrective action. Having verbs in our API endpoint paths isn’t useful and it makes it unnecessarily long since it doesn’t convey any new information.
OAuth 2.0 is an open standard for token-based authentication and authorization that is widely used in modern APIs. Another approach is to use JSON Web Tokens (JWTs) which provide a compact and secure way to transmit data between the client and the server. APIs (Application Programming Interfaces) are the linchpins of the software world, enabling different systems to communicate and work together. Delving into the realm of API design patterns uncovers a plethora of strategies crucial for crafting efficient, robust, and scalable APIs. Let's explore the various API design patterns, each accompanied by its own set of secrets that can transform the way you approach API development.
I'm using the shorthand syntax here, to create a new key called "mode" inside the object with the value of whatever is in "req.query.mode". This could be either a truthy value or undefined if there isn't a query parameter called "mode". We can extend this object the more filter parameters we'd like to accept. Now we're able to throw and catch errors in the service and data access layer. We can move into our workout controller now, catch the errors there as well, and respond accordingly.
RESTful APIs have become the standard for building web services that are scalable, flexible, and easy to maintain. However, building a successful RESTful API requires careful planning, implementation, and testing. In this answer, we'll cover some of the best practices for building a RESTful API, as well as some common pitfalls to avoid. Where kesh92 is the username of a specific user in the users collection, and will return the location and date of joining for kesh92. These are just some of the ways you could design parameters that strive towards API completion and help your end developers use your API intuitively.
Comments
Post a Comment